Appearance
Introduction
The Vim OS platform allows your application to become context-aware and tightly integrate into the workflow, in this section we’ll cover what context could be provided from the EHR to your app and what you’re app could write back into the EHR.
Note:
Note:
We will describe how you can move your current non-integrated to EHR generic application into an application that is bi-directional integrated with the most popular EHRs.
How does it work?
Once VimOS.js is integrated into your application, it will monitor the EHR resources you requested permissions for in your application manifest and share their associated data based on the users session with the EHR. It then abstracts that and provides a query interface for you as a developer to adjust your experience based on what’s currently happening at the point of care.
Read EHR resources
vimOS.ehr
is where you can leverage Vim's integration capabilities with the EHR, such as the ability to read/write within the EHR and enable workflow resources and their associated entity data.
vimOS.ehr.ehrState
holds the information on the current active session context for the EHR entities.
WARNING
Your application will only receive the information for those entities it declared read access for in the Developer Console; Entities for which read access wasn't declared will always be undefined
.
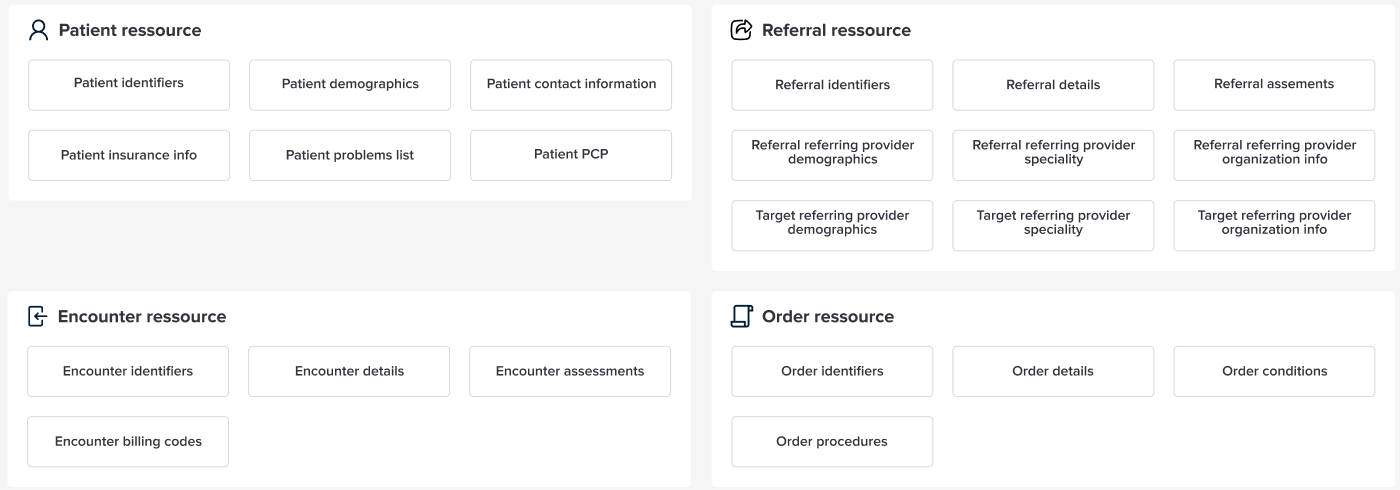
Subscribe to EHR resources
Once your application is integrated at the point of care, it is recommended that you subscribe to specific resources in the SDK. Doing so will keep you informed about any real-time changes or updates related to those resources, which will enable you to develop a more dynamic and responsive user experience.
To subscribe to any resource, call vimOS.ehr.subscribe(resource, callback)
.
Note:
TIP
- Your callback function will be called immediately with the current value of the resource, and will be called again whenever the resource is changed in the EHR.
- When you no longer want or need to receive updates, you should unsubscribe to avoid memory leaks:
vimOS.ehr.unsubscribe(resource, callback)
.
Example:
ts
import { EHR } from 'vim-os-js-browser/types';
const onPatientChange = (patient: EHR.Patient) => {
//do something with the updated patient
};
vimOS.ehr.subscribe(EHR.EHRResource.patient, onPatientChange);
...
vimOS.ehr.unsubscribe(EHR.EHRResource.patient, onPatientChange);
js
const onPatientChange = (patient) => {
//do something with the updated patient
}
vimOS.ehr.subscribe(EHR.EHRResource.patient, onPatientChange);
...
vimOS.ehr.unsubscribe(EHR.EHRResource.patient, onPatientChange);
Patient
Patient resources includes Information regarding personal details, administrative and clinical data of an individual receiving care or other health-related services.
It is available under vimOS.ehr.ehrState.patient
and holds the information about the Patient currently in context.
Patient
ts
interface Patient {
identifiers: {
ehrPatientId: string;
vimPatientId: string;
mrn?: string;
};
demographics: {
firstName: string;
lastName: string;
middleName?: string;
dateOfBirth: string; //ISO string, i.e yyyy-MM-dd
gender?: 'male' | 'female';
};
address?: {
address1?: string;
address2?: string;
city?: string;
state?: string;
zipCode?: string;
fullAddress?: string;
};
insurance?: {
ehrInsurance?: string;
groupId?: string;
payerId?: string;
memberId?: string;
};
contact_info?: {
homePhoneNumber?: string;
mobilePhoneNumber?: string;
email?: string;
};
pcp?: Provider;
};
ts
const patient = {
identifiers: {
ehrPatientId: '137931',
mrn: '12312312313' ,
vimPatientId: '123e4567-e89b-12d3-a456-426614174000' ,
},
demographics: {
firstName: 'Patricia',
lastName: 'Bell',
middleName: '' ,
dateOfBirth: '1950-09-06',
gender: 'female',
},
address: {
address1: '312 Walker Ave',
address2: '',
city: 'BROOKSVILLE',
state: 'FL',
zipCode: '34601',
fullAddress: '312 Walker Ave, BROOKSVILLE, FL, 34601',
},
insurance: {
ehrInsurance: 'Florida Blue',
groupId: '90',
payerId: '00590',
memberId: 'H32880421',
},
contact_info: {
homePhoneNumber: '212-123-1231',
mobilePhoneNumber: '123-123-1231' ,
email: '[email protected]',
},
pcp: {
EHRProviderId: '2342423',
npi: 1236677889,
demographics: {
firstName: 'John',
lastName: 'Doe',
middleName: 'Van',
},
facility: {
facilityEhrId: '132321',
name: 'Orchard Group',
address: {
address1: '6044 NORTH KEATING',
address2: '',
city: 'CHICAGO',
state: 'IL',
zipCode: '60646',
fullAddress: '6044 NORTH KEATING, CHICAGO, IL, 60646',
},
contact_info: {
mobilePhoneNumber: '212-222-333',
homePhoneNumber: '333-444-333',
faxNumber: '444-555-333',
email: '[email protected]',
},
},
specialty: 'Reproductive Endocrinology/Infertility',
providerDegree: 'Prof',
},
};
Patient Problem List
Rate limit
DANGER
You can send no more than 10 requests per minute.
To receive the Problem List for the Patient in context, use the getProblemList function on the Patient object:
ts
if (vimOS.ehr.ehrState?.patient) {
try {
const problemList = await vimSdk.ehr.ehrState.patient.getProblemList();
} catch (error) {
console.error('failed to load patient list', error);
}
}
If the patient has any problems, the returned problemList
will be an array of type Diagnosis
ts
type ProblemList = Diagnosis[] | undefined;
Encounter
An encounter refers to a meeting between a patient and healthcare provider(s) for providing healthcare services or evaluating the patient's health status. The term "encounter" is typically used to document activities in contrast to an appointment that notes planned activities.
It is available under vimOS.ehr.ehrState.encounter
which holds the information about the encounter currently in context.
Encounter
ts
interface Encounter {
ehrEncounterId?: string;
provider?: Provider;
assessments?: Diagnosis[];
status?: 'LOCKED' | 'UNLOCKED';
encounterDateOfService?: string;; // yyyy-MM-dd
};
ts
const encounter = {
ehrEncounterId: 'a399e79d-0bdd-4ca8-bfc9-65e7363bd283',
provider: {
EHRProviderId: '2342423',
npi: 1236677889,
demographics: {
firstName: 'John',
lastName: 'Doe',
middleName: 'Van',
},
facility: {
facilityEhrId: '132321',
name: 'Orchard Group',
address: {
address1: '6044 NORTH KEATING',
address2: '',
city: 'CHICAGO',
state: 'IL',
zipCode: '60646',
fullAddress: '6044 NORTH KEATING, CHICAGO, IL, 60646',
},
contact_info: {
mobilePhoneNumber: '212-222-333',
homePhoneNumber: '333-444-333',
faxNumber: '444-555-333',
email: '[email protected]',
},
},
specialty: 'Reproductive Endocrinology/Infertility',
providerDegree: 'Prof',
},
assessments: [{
code: 'E11.9',
system: 'ICD-10',
description: 'Type 2 diabetes mellitus without complications',
}],
status: 'LOCKED',
encounterDateOfService:'2023-03-18',
};
Referral
A referral in healthcare refers to directing a patient from one healthcare provider or facility to another, usually for specialized care or further evaluation. It's a formal request made by one healthcare provider to another, often initiated when the patient's condition requires expertise beyond the referring provider's scope. Referrals are documented instances indicating a need for additional medical attention or specialized services. In the context of the vimOS.ehr.ehrState.referral
, it holds information about the specific referral currently under consideration or in progress.
Referral
ts
interface Referral {
ehrReferralId?: string;
vimReferralId?: string;
specialty?: string;
diagnosis?: Diagnosis[];
targetProvider?: Provider;
referringProvider?: Provider;
startDate?: string; // yyyy-MM-dd
endDate?: string; // yyyy-MM-dd
createdDate?: string; // yyyy-MM-dd
status?: 'DRAFT' | 'SIGNED' | 'DELETED';
priority?: 'ROUTINE' | 'URGENT' | 'STAT';
numberOfVisits?: string;
cpts?: ProceduresCodes[];
facilityName?: string;
authCode?: string;
isLocked?: boolean;
reasons?: string[];
notes?: string;
};
ts
const referral = {
ehrReferralId: '123123231',
vimReferralId: '6f29cebe-6fd8-46dc-8a6e-6750cb9ca52a',
specialty: 'cardiology',
diagnosis: [{
code: 'E11.9',
system: 'ICD-10',
description: 'Type 2 diabetes mellitus without complications',
}],
targetProvider: {
EHRProviderId: '2342423',
npi: 1236677889,
demographics: {
firstName: 'John',
lastName: 'Doe',
middleName: 'Van',
},
facility: {
facilityEhrId: '132321',
name: 'Orchard Group',
address: {
address1: '6044 NORTH KEATING',
address2: '',
city: 'CHICAGO',
state: 'IL',
zipCode: '60646',
fullAddress: '6044 NORTH KEATING, CHICAGO, IL, 60646',
},
contact_info: {
mobilePhoneNumber: '212-222-333',
homePhoneNumber: '333-444-333',
faxNumber: '444-555-333',
email: '[email protected]',
},
},
specialty: 'Reproductive Endocrinology/Infertility',
providerDegree: 'Prof',
},
referringProvider: {
EHRProviderId: '12345',
npi: 11112223344,
demographics: {
firstName: 'Robert',
lastName: 'Don',
middleName: 'Redman',
},
facility: {
facilityEhrId: '112333',
name: 'Family Home',
address: {
address1: '1111 NORTH KEATING',
address2: '',
city: 'CHICAGO',
state: 'IL',
zipCode: '60646',
fullAddress: '1111 NORTH KEATING, CHICAGO, IL, 60646',
},
contact_info: {
mobilePhoneNumber: '212-222-333',
homePhoneNumber: '333-444-333',
faxNumber: '444-555-333',
email: '[email protected]',
},
},
specialty: 'family medicine',
providerDegree: 'Dr',
},
startDate: '2023-03-18'
endDate: '2023-08-18'
createdDate: '2023-03-18'
status: 'SIGNED'
priority: 'ROUTINE'
numberOfVisits: '6',
cpts: [{
code: 'M1008',
system: 'CPT-2',
description: '<50% total pt outpt ra encts',
}],
facilityName: 'Orange Mansion',
authCode: '12321234234',
status: 'signed',
reasons: 'text 123 123',
notes: 'text 123 123',
};
Orders
An order resource represents a formal request for an action to be carried out for the patient. It is expected to result in one or more responses detailing the outcome of processing or handling the order. The order resource primarily focuses on requesting an action, while the specific details of the action itself are described in a separate resource. Order, can be presented in the EHR as a unique screen with one order or a screen showing multiple orders and hence it is presented as an array.
vimOS.ehr.ehrState.orders
holds the information about the list of Orders currently in context.
Orders
ts
interface Orders {
ehrOrderId?: string;
type?: 'LAB' | 'DI' | 'PROCEDURE';
icd?: Diagnosis[];
cpts?: ProceduresCodes[];
ehrEncounterId?: string;
createdDate?: string; // YYYY-MM-DD
}[]
ts
const orders = [{
EHROrderId: '123131',
type: 'Lab'
icd: [{
code: 'E11.9',
system: 'ICD-10',
description: 'Type 2 diabetes mellitus without complications',
}],
cpts: [{
code: 'M1008',
system: 'CPT-2',
description: '<50% total pt outpt ra encts',
}],
EHREncounterId: '123123',
createdDate: '2023-03-18',
}];
Coming soon: Events
In an upcoming release, we will introduce new events for app developers to use, allowing you to listen to changes made to EHRs.
Events are a way to notify or signal that a specific user interaction has been completed within the EHR . You can leverage events to facilitate communication between EHRs and your application, allowing your application to react to changes or trigger actions based on those changes. Events are often implemented using the observer pattern, where one part of the code (the observer) registers interest in certain events and provides a callback function to be executed when those events occur. When the event occurs, the SDK notifies all registered observers by calling their callback functions. For example, you will have events that notify your application when an encounter is opened, a referral is saved and so on. Your application can then listen for these events and update its interface or perform other actions accordingly.
Stay tuned for more details on the new EHR events and how you can integrate them into your applications to enhance the user experience.
Writeback to EHR resources
You can change values in the EHR using vimOS.ehr.resourceUpdater
.
WARNING
To update a specific EHR entity you must declare write access
for the entity in the Developer Console.
Rate limit
DANGER
You can send no more than 10 update requests per minute per user session.
A writeback to the EHR refers to adding information to the patient's electronic health record system from your external application. This functionality interacts with the EHR and contributes new data to the patient's record.
For example, a healthcare application might use writeback functionality to add a new assessment on encounter, a target provider on referral and so on. Writeback capabilities allow you to increase the efficiency and accuracy of the clinician user and are essential for ensuring that the patient's record is accurate and up-to-date across all systems that access it.
Resource Update API
The resource update API provides methods for updating specific aspects within the EHR.
Best Practices for Using the Resource Update API
TIP
The availability of the Resource Update API is influenced by various factors, such as the user's current screen, app permissions, and specific EHR dependencies. For example, certain fields may not be supported by the Vim infrastructure or the EHR itself. To identify the fields that are available for writeback for your application at any given time, we suggest using our canUpdate API.
Update Methods
These methods facilitate the updating of specific resource fields within the EHR. Certain input fields will override existing EHR data, while others will be appended to it (see the details in the interfaces sections below). The entire update payload is optional, allowing for flexibility in the update process.
Encounter
A subset of fields within the encounter entity can be modified with the updateEncounter
function. This asynchronous method resolves once the operation has been completed.
ts
updateEncounter(params: UpdateEncounterParams): Promise<void>
type UpdateEncounterParams = {
subjective?: {
// Appends input string into current encounter subjective General notes field.
generalNotes?: SafeText;
// Appends input string into current encounter subjective CC notes field.
chiefComplaintNotes?: SafeText;
// Appends input string into current encounter subjective HPI notes field.
historyOfPresentIllnessNotes?: SafeText;
// Appends input string into current encounter subjective ROS notes field .
reviewOfSystemsNotes?: SafeText;
};
objective?: {
// Appends input string into current encounter notes.
generalNotes?: SafeText;
// Appends input string into current encounter notes.
physicalExamNotes?: SafeText;
};
assessments?: {
// Appends input string into current encounter assessments general notes field.
generalNotes?: SafeText;
// Adds ICD codes input array into current encounter list.
diagnosisCodes?: UpdatableDiagnosis[];
};
plans?: {
// Appends input string into current encounter plans general notes field.
generalNotes?: SafeText;
// Adds procedures codes input array into current encounter list.
procedureCodes?: UpdatableProcedures[];
};
patientInstructions?: {
// Appends input string into current encounter patient instructions general notes field.
generalNotes?: SafeText;
};
};
Referral
A subset of fields within the referral entity can be modified using the updateReferral
function. This asynchronous method resolves once the operation has been completed.
ts
updateReferral(params: UpdateReferralParams): Promise<void>
type UpdateReferralParams = {
// Appends input string into current referral notes.
notes?: SafeText;
// Appends input string array into current referral reasons.
reasons?: SafeText[];
// Appends input string into current referral authCode.
authCode?: string;
// Overrides referral specialty with input string.
specialty?: string;
// Overrides referral startDate with input string date.
startDate?: string;
// Overrides referral endDate with input string date.
endDate?: string;
// Overrides referral priority with input enum.
priority?: ReferralPriority;
// Overrides referral diagnosis.
diagnosis?: UpdatableDiagnosis[];
// Overrides referral cpts.
cpts?: UpdatableProcedures[];
// Overrides referral numberOfVisits.
numberOfVisits?: string;
// Overrides referral targetProvider.
targetProvider?: UpdatableProvider;
};
Safe String
Some of the writeback fields have a type of SafeText
.
SafeText
is a string below 60K characters, containing only English characters, numbers, and the following special characters: ~
, `, !
, @
, #
, $
, %
, ^
, &
, *
, (
, )
, -
, _
, +
, =
, {
, }
, [
, ]
, |
, \
, ;
, :
, '
, "
, ,
, <
, >
, .
, /
, ?
, along with whitespace, newline, tab, and carriage return, and not containing any of the character sequences &
, <
, >
, "
, &apos
, &#
, ]]
.
More specifically, we validate the input by verifying that it doesn't include any of the sequences &
, <
, >
, "
, &apos
, &#
, ]]
, and that it matches the regex pattern
^[a-zA-Z 0-9~`!@#$%^&*()-_+={[}\]|\\;:'",<>.\/?\n\t\r]+$
Error Handling
In the event that at least one of the payload fields fails to update in the EHR, the methods will throw an error. Some fields might have succeeded, and it is the application's responsibility to notify the user appropriately of this inconsistent state.
Other scenarios where the operation may result in an error include validation issues, timeouts, and attempting to update fields that are not supported (refer to the next section for further details).
Error Type | Description |
---|---|
internal_error | Some fields might have successfully updated, but an internal error occurred. |
validation_error | The payload is invalid; writeback is blocked. |
authorization_error | The application does not have the necessary permissions; writeback is blocked. |
rate_limit_error | The rate limit has been exceeded; writeback is blocked. |
In case of an error, the writeback promise will reject with the following object:
ts
type ResourceUpdateError = {
type: 'internal_error' | 'validation_error' | 'authorization_error' | 'rate_limit_error',
data: any,
}
Long Execution
The EHR update operation may take up to one minute to execute. Vim-Os manages these scenarios by preventing editing of the EHR and displaying a loading overlay on the screen.
Can Update Methods
Important To Note
TIP
We recommend always calling the canUpdate function before performing a writeback. This helps to better understand the current availability of fields and ensures that the writeback is only attempted when relevant, thereby avoiding errors.
The availability of the Resource Update API is influenced by various factors, such as the user's current screen, app permissions, and specific EHR dependencies. For example, certain fields may not be supported by the Vim infrastructure or the EHR itself. To identify the fields that are available for writeback for your application at any given time, we suggest using our canUpdate
function.
This function does not validate the input values of the payload. Instead, it checks the validity of the operation itself at a given point in time, based on the fields set to be updated. The validation of the input values happens as part of the update function and will result in errors if the values are invalid.
When using canUpdate API, you should send only the relevant fields intended for writeback from your app. This approach ensures that you receive responses tailored to your specific requirements.
Encounter
This method checks whether an encounter can be updated based on the provided input fields. It receives a payload containing the fields that we intend to update.
ts
canUpdateEncounter(params: CanUpdateEncounterParams): CanUpdateEncounterResult
type CanUpdateEncounterParams = {
subjective?: {
generalNotes?: boolean;
chiefComplaintNotes?: boolean;
historyOfPresentIllnessNotes?: boolean;
reviewOfSystemsNotes?: boolean;
};
objective?: {
generalNotes?: boolean;
physicalExamNotes?: boolean;
};
assessments?: {
generalNotes?: boolean;
diagnosisCodes?: boolean;
};
plans?: {
generalNotes?: boolean;
procedureCodes?: boolean;
};
patientInstructions?: {
generalNotes?: boolean;
};
};
type CanUpdateEncounterResult = {
/*
* If at least one of the requested fields is unavailable,
* the value will be false.
*/
canUpdate: boolean;
/*
* Contains boolean flags indicating the fields you want to check for update availability,
* but with values set to false for any fields that cannot be updated.
*/
details: CanUpdateEncounterParams
};
Referral
This method checks whether a referral can be updated based on the provided input fields. It receives a payload containing the fields that we intend to update.
ts
canUpdateReferral(params: CanUpdateReferralParams): CanUpdateReferralResult
type CanUpdateReferralParams = {
notes?: boolean;
reasons?: boolean;
authCode?: boolean;
specialty?: boolean;
startDate?: boolean;
endDate?: boolean;
priority?: boolean;
diagnosis?: boolean;
cpts?: boolean;
numberOfVisits?: boolean;
targetProvider?: boolean;
};
type CanUpdateReferralResult = {
/*
* If at least one of the requested fields is unavailable,
* the value will be false.
*/
canUpdate: boolean;
/*
* Contains boolean flags indicating the fields you want to check for update availability,
* but with values set to false for any fields that cannot be updated.
*/
details: CanUpdateReferralParams
};
Code Examples
1. Check if canUpdateEncounter, then updateEncounter
In the below code snippet that we:
- Want to update the
diagnosisCodes
, theprocedureCodes
, and both theassessments.generalNotes
and theplans.generalNotes
fields of the encounter- In case the
plans.generalNotes
is not updatable (which may very well be the case in some EHR systems), we updateassessments.generalNotes
with the joined text
- In case the
- Initiate the encounter writeback process.
- Handle errors that occur in the event of an update failure.
updateEncounter
js
const canUpdateRequiredFields = vimOS.ehr.resourceUpdater.canUpdateEncounter({
assessments: {
generalNotes: true,
diagnosisCodes: true,
},
plans: {
procedureCodes: true,
}
});
const canUpdatePlansNotes = vimOS.ehr.resourceUpdater.canUpdateEncounter({
plans: {
generalNotes: true,
}
});
const assessmentsGeneralNotes = "text to add to assessments generalNotes";
const plansGeneralNotes = "text to add to plans generalNotes";
if (canUpdateRequiredFields.canUpdate) {
try {
await vimOS.ehr.resourceUpdater.updateEncounter({
assessments: {
generalNotes: canUpdatePlansNotes.canUpdate ? assessmentsGeneralNotes : assessmentsGeneralNotes + plansGeneralNotes,
diagnosisCodes: [{
code: 'H02.403',
description: 'Unspecified hypothyroidism with diffuse goiter without thyrotoxic crisis'
}],
},
plans: {
procedureCodes: [{
code: '2035F',
description: 'Tympanic membrane mobility assessed with pneumatic otoscopy or tympanometry'
}],
generalNotes: canUpdatePlansNotes.canUpdate ? plansGeneralNotes : undefined,
},
});
// show success message to the user.
} catch (error) {
// show message to the user indicating that something went wrong.
}
} else {
// show message to the user if can't update...
}
2. Check if canUpdateReferral, then updateReferral
In this example we:
- Check if certain referral fields of the EHR are writable at this point.
- Initiate the referral writeback process.
- Handle errors that occur in the event of an update failure.
updateReferral
js
const res = vimOS.ehr.resourceUpdater.canUpdateReferral({
diagnosis: true,
authCode: true,
});
if (res.canUpdate) {
try {
await vimOS.ehr.resourceUpdater.updateReferral({
diagnosis: [{
code: 'H02.403',
description: 'Unspecified hypothyroidism with diffuse goiter without thyrotoxic crisis'
}],
authCode: 'RFA123456'
});
// show success message to the user.
} catch (error) {
// show message to the user indicating that something went wrong.
}
} else {
// show message to the user...
}
Common types
A type refers to the kind of data that a variable or expression can hold or produce.
Diagnosis codes
Diagnosis codes in healthcare are alphanumeric codes used to represent specific medical diagnoses or conditions. These codes are part of a standardized system, such as the International Classification of Diseases (ICD-10), and are used by healthcare providers and insurance companies for billing, reimbursement, and statistical purposes.
Each diagnosis code corresponds to a particular medical condition, symptom, or disease, allowing for accurate documentation and communication within the healthcare system.
Diagnosis
type holds the information about the diagnosis code details that are available and can be part of the encounter, referral state for example.
Diagnosis
ts
interface Diagnosis {
code: string;
system: string;
description?: string;
};
ts
const diagnosis = {
code: 'E11.9',
system: 'ICD-10',
description: 'Type 2 diabetes mellitus without complications',
};
Procedure codes
Procedure codes in healthcare are alphanumeric codes used to represent specific medical procedures, treatments, or services provided to patients. Like diagnosis codes, procedure codes are part of standardized systems like the Current Procedural Terminology (CPT-II) or the Healthcare Common Procedure Coding System (HCPCS). These codes facilitate billing, reimbursement, and tracking of medical services rendered.
ProceduresCodes
type holds the information about the procedure code details available that can be part of the order or referral state for example.
ProceduresCodes
ts
interface ProceduresCodes {
code: string;
system: string;
description?: string;
};
ts
const proceduresCodes = {
code: 'M1008',
system: 'CPT-2',
description: '<50% total pt outpt ra encts',
};
Provider
A provider in healthcare refers to an individual or entity that delivers medical services or care to patients. This could include physicians, nurses, therapists, clinics, hospitals, or other healthcare professionals or institutions. Providers play a crucial role in delivering healthcare services and are often responsible for diagnosing and treating patients, prescribing medications, performing procedures, and coordinating care. In the context of the SDK documentation, details about providers are available through the provider type and can be part of the encounter, referral or other state.
Provider
type holds the information about the provider details available that can be part of the encounter or referral state, for example.
Provider
ts
interface Provider {
ehrProviderId: string;
npi?: string;
demographics: {
firstName: string;
lastName: string;
middleName?: string;
};
facility?: {
facilityEhrId?: string;
name?: string;
address?: {
address1?: string;
address2?: string;
city?: string;
state?: string;
zipCode?: string;
fullAddress?: string;
};
contact_info?: {
mobilePhoneNumber?: string;
homePhoneNumber?: string;
faxNumber?: string;
email?: string;
};
};
specialty?: string[];
providerDegree?: string;
};
ts
const provider = {
EHRProviderId: '2342423',
npi: 1236677889,
demographics: {
firstName: 'John',
lastName: 'Doe',
middleName: 'Van',
},
facility: {
facilityEhrId: '132321',
name: 'Orchard Group',
address: {
address1: '6044 NORTH KEATING',
address2: '',
city: 'CHICAGO',
state: 'IL',
zipCode: '60646',
fullAddress: '6044 NORTH KEATING, CHICAGO, IL, 60646',
},
contact_info: {
mobilePhoneNumber: '212-222-333',
homePhoneNumber: '333-444-333',
faxNumber: '444-555-333',
email: '[email protected]',
},
},
specialty: 'Reproductive Endocrinology/Infertility',
providerDegree: 'Prof',
}